Understanding Python Module Packaging: A Hands-on Guide to.whl File Packaging
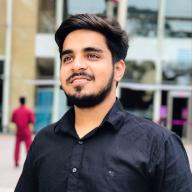
Table of Contents
What are .whl Files? #
Wheels are the new standard of Python distribution and are intended to replace eggs. Support is offered in pip >= 1.4 and setuptools >= 0.8.1
A Wheel, or .whl
file, is a binary distribution format designed specifically for use with
Python applications. Wheels, as contrast to conventional source distributions, come with
precompiled code, which makes deployments quicker and more effective.
How to Create Wheel Files? #
Step 1: Setup the Environment #
Make sure you have the necessary tools installed before you start packing. Execute the subsequent command:
pip install wheel setuptools
Step 2: Create setup.py #
Make a setup.py file in your project’s root directory to include package metadata. Observe this simple example:
from setuptools import setup, find_packages
setup(
name='your_package_name',
version='0.1.0',
packages=find_packages(),
install_requires=[
# List your dependencies here
],
)
Optional Stuff in setup.py
#
Here are some additional options you can include in the setup()
function:
- Packages
packages=['your_package_name', 'your_package_name.submodule']
- Package_data
package_data={'your_package_name': ['data/*.csv', 'config/*.ini'],}
- Include_package_data:
include_package_data=True
- Zip_safe:
zip_safe=False
- Python_requires:
python_requires='>=3.6'
- Author and Author’s Email:
author='YourName', author_email='your.email@example.com'
- License:
license='MIT'
- Packages that are required to be installed for your package to work correctly:
install_requires=[ 'dependency1>=1.0', 'dependency2==2.3.4', ]
Step 3: Create init.py file #
Create a folder and create a __init__.py
file in that folder and write your class, module which
you want to access when you install your .whl
file.
Step 4: Construct the Wheel Distribution #
Run the following command to create the distribution of wheels:
python setup.py sdist bdist_wheel
After running the above code we will get these two files in dist Folder:
dist
|-- demopack-0.1.tar.gz
|-- demopack-0.1-py3-none-any.whk
Step 5: Distribute Your Box #
Give people access to the.whl file you produced. Installing it may be done with:
pip install your_package_name-0.1.0-py3-none-any.whl
Step 6: If desired, upload to PyPI (Optional) #
You might think about submitting your package to the Python Package Index (PyPI) for wider distribution. After installing twine, do the below commands:
pip install twine
twine upload dist/*
You may need to create an account on pypi for this before.
Conclusion #
Being able to package your Python modules into.whl files improves your ability to contribute to the open-source Python community. Wheels provide a smooth integration experience for your code into a variety of applications with faster installations, broad compatibility, and easier dependency management. Take pride in your packaging, spread the word about your inventions, and contribute significantly to the growing Python community